mirror of
https://github.com/st-tu-dresden-praktikum/swt23w23
synced 2024-07-19 21:04:36 +02:00
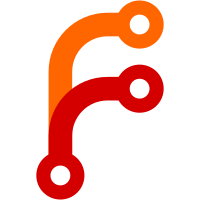
Works on #73 This contains changes on multiple files to associate multiple employees with an order and make customers able to add employees to their order. Because of SQL JPA things, this will not be final.
198 lines
6.4 KiB
Java
198 lines
6.4 KiB
Java
package catering.order;
|
|
|
|
import catering.staff.JobType;
|
|
import catering.staff.Staff;
|
|
import catering.staff.StaffManagement;
|
|
import org.junit.jupiter.api.BeforeEach;
|
|
import org.junit.jupiter.api.Test;
|
|
import org.salespointframework.catalog.Product;
|
|
import org.salespointframework.inventory.UniqueInventory;
|
|
import org.salespointframework.inventory.UniqueInventoryItem;
|
|
import org.salespointframework.order.CartItem;
|
|
import org.salespointframework.order.OrderManagement;
|
|
import org.salespointframework.quantity.Quantity;
|
|
import org.salespointframework.useraccount.Password;
|
|
import org.salespointframework.useraccount.Role;
|
|
import org.salespointframework.useraccount.UserAccount;
|
|
import org.salespointframework.useraccount.UserAccountManagement;
|
|
import org.springframework.beans.factory.annotation.Autowired;
|
|
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
|
|
import org.springframework.boot.test.context.SpringBootTest;
|
|
import org.springframework.data.domain.Pageable;
|
|
import org.springframework.security.test.context.support.WithMockUser;
|
|
import org.springframework.test.web.servlet.MockMvc;
|
|
|
|
import static org.hamcrest.Matchers.not;
|
|
import static org.springframework.security.test.web.servlet.request.SecurityMockMvcRequestPostProcessors.user;
|
|
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
|
|
import static org.hamcrest.CoreMatchers.containsString;
|
|
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.post;
|
|
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
|
|
|
|
import java.time.LocalDateTime;
|
|
|
|
|
|
@AutoConfigureMockMvc
|
|
@SpringBootTest
|
|
public class OrderControllerIntegrationTests {
|
|
|
|
@Autowired
|
|
MockMvc mvc;
|
|
|
|
@Autowired
|
|
UniqueInventory<UniqueInventoryItem> inventory;
|
|
|
|
@Autowired
|
|
OrderManagement<CustomOrder> orderManagement;
|
|
|
|
@Autowired
|
|
UserAccountManagement userAccountManagement;
|
|
|
|
@Autowired
|
|
StaffManagement staffManagement;
|
|
|
|
UserAccount myUser;
|
|
UserAccount admin;
|
|
CustomCart myCart;
|
|
Staff myStaff;
|
|
|
|
@BeforeEach
|
|
void setUp() {
|
|
if (userAccountManagement.findByUsername("andi").isEmpty()) {
|
|
userAccountManagement.create("andi",
|
|
Password.UnencryptedPassword.of("12345"), Role.of("CUSTOMER"));
|
|
}
|
|
|
|
myStaff = new Staff("Sabrina", JobType.SERVICE);
|
|
staffManagement.addStaff(myStaff);
|
|
|
|
myUser = userAccountManagement.findByUsername("andi").get();
|
|
admin = userAccountManagement.findByUsername("admin").get();
|
|
|
|
|
|
if (orderManagement.findAll(Pageable.unpaged()).stream().findAny().isEmpty()) {
|
|
myCart = new CustomCart(OrderType.EVENT_CATERING,
|
|
LocalDateTime.of(2023, 12, 23, 10, 0),
|
|
LocalDateTime.of(2023, 12, 24, 10, 0));
|
|
myCart.addOrUpdateItem(inventory.findAll().stream().findFirst().get().getProduct(), Quantity.of(1));
|
|
|
|
CustomOrder myOrder = new CustomOrder(myUser.getId(), myCart);
|
|
myCart.addItemsTo(myOrder);
|
|
|
|
orderManagement.payOrder(myOrder);
|
|
orderManagement.completeOrder(myOrder);
|
|
}
|
|
}
|
|
|
|
@Test
|
|
void customerViewsOrder() throws Exception {
|
|
mvc.perform(get("/myOrders").with(user("andi").roles("CUSTOMER")))
|
|
.andExpect(status().isOk())
|
|
.andExpect(content().string(containsString("Menge")))
|
|
.andExpect(model().attributeExists("orders"));
|
|
|
|
mvc.perform(get("/myOrders").with(user("admin").roles("CUSTOMER"))) // WTF SPRING!11!!!!
|
|
.andExpect(status().isOk())
|
|
.andExpect(content().string(containsString("0")));
|
|
|
|
mvc.perform(get("/myOrders").with(user("admin").roles("ADMIN")))
|
|
.andExpect(status().isForbidden());
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "admin", roles = "ADMIN")
|
|
void adminViewsOrder() throws Exception {
|
|
mvc.perform(get("/allOrders"))
|
|
.andExpect(status().isOk())
|
|
.andExpect(content().string(containsString("Menge")))
|
|
.andExpect(model().attributeExists("orders"));
|
|
|
|
mvc.perform(get("/allOrders").with(user("andi").roles("CUSTOMER")))
|
|
.andExpect(status().isForbidden());
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "admin", roles = "ADMIN")
|
|
void adminViewsOrderByDate() throws Exception {
|
|
mvc.perform(get("/allOrders/2023-12-23"))
|
|
.andExpect(status().isOk())
|
|
.andExpect(content().string(containsString("Menge")))
|
|
.andExpect(model().attributeExists("orders"));
|
|
|
|
mvc.perform(get("/allOrders/2023-10-12"))
|
|
.andExpect(status().isOk())
|
|
.andExpect(content().string(not(containsString("Menge"))))
|
|
.andExpect(model().attributeExists("orders"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "admin", roles = "ADMIN")
|
|
void adminRemovesOrder() throws Exception {
|
|
mvc.perform(post("/allOrders/remove")
|
|
.param("orderID", orderManagement.findAll(Pageable.unpaged()).stream().findFirst().get().getId().toString()))
|
|
.andExpect(status().is3xxRedirection())
|
|
.andExpect(redirectedUrl("/allOrders"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "andi", roles = "CUSTOMER")
|
|
void userPlansEvent() throws Exception {
|
|
mvc.perform(get("/event"))
|
|
.andExpect(status().isOk())
|
|
.andExpect(model().attributeExists("invItems"));
|
|
|
|
Product product = inventory.findAll().stream().findFirst().get().getProduct();
|
|
|
|
mvc.perform(post("/event/addProduct")
|
|
.param("pid", product.getId().toString())
|
|
.param("number", "2"))
|
|
.andExpect(status().is3xxRedirection())
|
|
.andExpect(redirectedUrl("/event"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "andi", roles = "CUSTOMER")
|
|
void userRemovesItemFromCart() throws Exception {
|
|
CartItem myItem = myCart.toList().get(0);
|
|
|
|
mvc.perform(post("/event/removeProduct")
|
|
.param("itemId", myItem.getId()))
|
|
.andExpect(status().is3xxRedirection())
|
|
.andExpect(redirectedUrl("/event"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "andi", roles = "CUSTOMER")
|
|
void userChangesDate() throws Exception {
|
|
mvc.perform(post("/event/changeDate")
|
|
.param("startDate", "2024-10-10")
|
|
.param("startHour", "10")
|
|
.param("finishDate", "2024-10-11")
|
|
.param("finishHour", "11"))
|
|
.andExpect(status().is3xxRedirection())
|
|
.andExpect(redirectedUrl("/event"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "andi", roles = "CUSTOMER")
|
|
void userCheckOut() throws Exception {
|
|
Product product = inventory.findAll().stream().findFirst().get().getProduct();
|
|
mvc.perform(post("/event/addProduct")
|
|
.param("pid", product.getId().toString())
|
|
.param("number", "2"));
|
|
|
|
mvc.perform(post("/event/checkout"))
|
|
.andExpect(status().is3xxRedirection())
|
|
.andExpect(redirectedUrl("/event"));
|
|
}
|
|
|
|
@Test
|
|
@WithMockUser(username = "andi", roles = "CUSTOMER")
|
|
void addStaff() throws Exception {
|
|
mvc.perform(post("/event/addStaff")
|
|
.param("sid", myStaff.getId().toString()))
|
|
.andExpect(redirectedUrl("/event"));
|
|
}
|
|
|
|
}
|